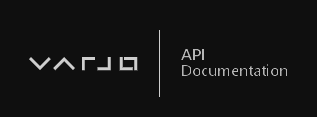 |
Varjo Native SDK
|
|
Go to the documentation of this file.
9 #include "Varjo_version_defines.h"
11 #if defined __cplusplus
578 #if defined __cplusplus
int64_t varjo_Nanoseconds
Time in nanoseconds.
Definition: Varjo_types.h:140
struct varjo_Event * varjo_AllocateEvent()
Allocates an event.
int32_t varjo_GetPropertyCount(struct varjo_Session *session)
Gets the number of system properties.
int32_t varjo_GetPropertyInt(struct varjo_Session *session, varjo_PropertyKey propertyKey)
Gets an integer value of a property.
void varjo_GetTextureSize(struct varjo_Session *session, varjo_TextureSize_Type type, int32_t viewIndex, int32_t *width, int32_t *height)
Gets texture size for specific varjo_TextureSize_type and view index.
struct varjo_Matrix varjo_ApplyTransform(struct varjo_Session *session, struct varjo_Matrix *m1, struct varjo_Matrix *m2)
Applies transform using post multiplication.
void varjo_FreeEvent(struct varjo_Event *event)
Free an event.
void varjo_GetPropertyString(struct varjo_Session *session, varjo_PropertyKey propertyKey, char *buffer, uint32_t maxSize)
Gets a string value of a property.
void varjo_FreeFrameInfo(struct varjo_FrameInfo *frameInfo)
Frees an allocated frame info.
void varjo_GazeInitWithParameters(struct varjo_Session *session, struct varjo_GazeParameters *parameters, int32_t parameterCount)
Initializes the gaze tracking system with provided parameters.
Parameters passed to varjo_GazeInitWithParameters function.
Definition: Varjo_types.h:463
View description.
Definition: Varjo_types.h:403
int64_t varjo_PoseType
Definition: Varjo_types.h:189
void varjo_D3D12UpdateVariableRateShadingTexture(struct varjo_Session *session, struct ID3D12GraphicsCommandList *commandList, struct ID3D12Resource *texture, struct varjo_VariableRateShadingConfig *config)
Creates variable rate shading texture.
struct varjo_Ray gaze
Normalized gaze direction ray.
Definition: Varjo_types.h:416
int64_t varjo_RenderAPI
Render API flags.
Definition: Varjo_types.h:226
int32_t varjo_Bool
Definition: Varjo_types.h:133
Gaze tracker vectors and tracking state.
Definition: Varjo_types.h:413
varjo_Bool varjo_GetPropertyBool(struct varjo_Session *session, varjo_PropertyKey propertyKey)
Gets a boolean value of a property.
void varjo_ResetPose(struct varjo_Session *session, varjo_Bool position, varjo_RotationReset rotation)
Resets pose tracking origin.
varjo_Nanoseconds varjo_GetCurrentTime(struct varjo_Session *session)
Gets the current time in a format that Varjo API uses.
int32_t varjo_GetViewCount(struct varjo_Session *session)
Gets numbers of views.
void varjo_SyncProperties(struct varjo_Session *session)
Updates and synchronizes system properties with the system state.
int64_t varjo_WindingOrder
Definition: Varjo_types.h:202
void varjo_GetSupportedTextureFormats(struct varjo_Session *session, varjo_RenderAPI renderApi, int32_t *formatCount, varjo_TextureFormat *formats)
Gets supported texture formats in the order of most preferred to least preferred.
const char * varjo_GetErrorDesc(varjo_Error error)
Gets error description for the specified error code.
Definition: Varjo_types.h:483
varjo_Bool varjo_HasProperty(struct varjo_Session *session, varjo_PropertyKey propertyKey)
Checks if the key has value in the properties.
struct varjo_AlignedView varjo_GetAlignedView(double *projectionMatrix)
Gets an aligned view from a projection matrix.
int64_t varjo_PropertyKey
System status properties.
Definition: Varjo_types.h:173
struct varjo_Matrix varjo_FrameGetPose(struct varjo_Session *session, varjo_PoseType type)
Gets a pose for the current frame.
void varjo_GazeInit(struct varjo_Session *session)
Initializes the gaze tracking system.
varjo_PropertyKey varjo_GetPropertyKey(struct varjo_Session *session, int32_t index)
Gets a property key for a property index.
varjo_Nanoseconds varjo_FrameGetDisplayTime(struct varjo_Session *session)
Gets the time when the frame is scheduled to be displayed.
Per-frame information.
Definition: Varjo_types.h:393
struct varjo_Session * varjo_SessionInit(void)
Initializes a Varjo client session.
const char * varjo_GetPropertyName(struct varjo_Session *session, varjo_PropertyKey propertyKey)
Gets the name of the property key.
Axis aligned tangents from a projection matrix.
Definition: Varjo_types.h:371
varjo_Bool varjo_GetRenderingGaze(struct varjo_Session *session, struct varjo_Gaze *gaze)
Returns gaze which can be used for foveated rendering or to generate variable rate shading texture.
Minimum and maximum limits for swap chain texture count and size.
Definition: Varjo_types.h:431
struct varjo_Gaze varjo_GetGaze(struct varjo_Session *session)
Gets the current state of the user gaze.
struct varjo_FrameInfo * varjo_CreateFrameInfo(struct varjo_Session *session)
Creates a frame info with initial values.
int64_t varjo_TextureFormat
Definition: Varjo_types.h:206
Event struct used for all the events by the event system.
Definition: Varjo_events.h:117
uint32_t varjo_GetPropertyStringSize(struct varjo_Session *session, varjo_PropertyKey propertyKey)
Gets the size of a buffer that is big enough to hold the property, including the null terminator.
void varjo_SessionSetPriority(struct varjo_Session *session, int32_t priority)
Sets session overlay priority.
Parameters passed to varjo_RequestGazeCalibrationWithParameters function.
Definition: Varjo_types.h:471
struct varjo_Matrix varjo_GetRelativePoseTransform(struct varjo_Session *session, varjo_PoseType src, varjo_PoseType dest)
Gets a relative transformation from source pose to destination pose.
varjo_Bool varjo_IsGazeAllowed(struct varjo_Session *session)
Is gaze allowed to be used?
struct varjo_Mesh2Df * varjo_CreateOcclusionMesh(struct varjo_Session *session, int32_t viewIndex, varjo_WindingOrder windingOrder)
Creates the occlusion mesh for a given view index.
struct varjo_Matrix varjo_GetTrackingToLocalTransform(struct varjo_Session *session)
Transform from tracking space to local client space.
struct varjo_ViewDescription varjo_GetViewDescription(struct varjo_Session *session, int32_t viewIndex)
Gets the display information for a given view.
const char * varjo_GetVersionString()
Gets the short version of the runtime.
varjo_Error varjo_GetError(struct varjo_Session *session)
Gets the latest error code.
varjo_Bool varjo_IsAvailable()
Checks whether Varjo system is available.
Double precision 4x4 matrix.
Definition: Varjo_types.h:293
void varjo_RequestGazeCalibration(struct varjo_Session *session)
Requests a HMD gaze calibration.
varjo_Bool varjo_PollEvent(struct varjo_Session *session, struct varjo_Event *evt)
Polls events.
Definition: Varjo_types.h:510
int64_t varjo_Error
Definition: Varjo_types.h:13
struct varjo_FovTangents varjo_GetFovTangents(struct varjo_Session *session, int32_t viewIndex)
Returns tangents for a given view index.
double varjo_GetPropertyDouble(struct varjo_Session *session, varjo_PropertyKey propertyKey)
Gets a floating point value of a property.
int64_t varjo_RotationReset
Rotation reset types.
Definition: Varjo_types.h:165
struct varjo_SwapChainLimits varjo_GetSwapChainLimits(struct varjo_Session *session)
Gets swap chain limits.
void varjo_SetCenteredProjection(struct varjo_Session *session, varjo_Bool enabled)
Forces the provided projection matrices to be centered.
void varjo_FreeOcclusionMesh(struct varjo_Mesh2Df *mesh)
Frees the memory allocated by varjo_CreateOcclusionMesh.
const uint64_t varjo_GetVersion()
Gets the version number of the runtime.
struct varjo_FovTangents varjo_GetFoveatedFovTangents(struct varjo_Session *session, int32_t indexView, struct varjo_Gaze *gaze, struct varjo_FoveatedFovTangents_Hints *hints)
Returns foveated view tangents for a given index and gaze vector.
void varjo_SessionShutDown(struct varjo_Session *session)
Shuts down a session and releases all the resources internally allocated by Varjo session.
int32_t varjo_GetGazeArray(struct varjo_Session *session, struct varjo_Gaze *array, int32_t maxSize)
Gets gaze measurements since last query.
2D triangle list mesh.
Definition: Varjo_types.h:360
void varjo_WaitSync(struct varjo_Session *session, struct varjo_FrameInfo *frameInfo)
Called at the start of a frame.
Definition: Varjo_types.h:490
void varjo_RequestGazeCalibrationWithParameters(struct varjo_Session *session, struct varjo_GazeCalibrationParameters *parameters, int32_t parameterCount)
Requests a HMD gaze calibration with provided parameters.
int64_t varjo_TextureSize_Type
Definition: Varjo_types.h:476